Two-ion Vibrationally Assisted Energy Transport (VAET)
Contents
Two-ion Vibrationally Assisted Energy Transport (VAET)#
using QuantumOptics
using IonSim
import PyPlot
const plt = PyPlot;
# set some plot configs
plt.matplotlib.rc("xtick", top=false)
plt.matplotlib.rc("ytick", right=false, left=false)
plt.matplotlib.rc("axes", labelsize=20, titlesize=20, grid=true)
plt.matplotlib.rc("axes", linewidth=2)
plt.matplotlib.rc("grid", alpha=0.25, linestyle="--")
plt.matplotlib.rc("font", family="Palatino", weight="medium")
plt.matplotlib.rc("figure", figsize=(8,4))
plt.matplotlib.rc("xtick.major", width=2)
plt.matplotlib.rc("ytick.major", width=2)
Background#
In [GHM+18] it is demonstrated that two ions in a Paul trap can be used to simulate the following Hamiltonian:
whose dynamics are capable of exhibiting a phenomenon known as vibrationally assisted energy transport (VAET).
For an initial state \(|\uparrow \downarrow\rangle\), the effect of \(\hat{H}_{S-S}\) is to drive population exchange between the states \(|\uparrow \downarrow\rangle \leftrightarrow |\downarrow \uparrow\rangle\) at a rate \(J/2\).
\(\hat{H}_{S}\) acts as an energy barrier to this exchange with a strength \(\Delta\). If \(\Delta\) becomes comparable in magnitude to \(J\), then population exchange will be highly suppressed.
\(\hat{H}_{S-B}\) couples the 2\(^{nd}\) spin to the vibrational mode – whose self-energy is described by \(\hat{H}_{B}\). Even when the energy barrier to spin transitions is large (\(\Delta \gg J\)), if also \(\nu_{eff} \approx \Delta\), then population transfer between the spins can still be achieved by effectively borrowing or storing energy in the coupled vibrational mode. This is the basis of VAET.
In this notebook, we do a step-by-step walkthrough on how to simulate this effect using IonSim.
\(\hat{H}_{S-S}\)#
Engineering the spin-spin coupling term#
In the lab, \(\hat{H}_{S-S}\) can be engineered with two laser tones, using a Mølmer-Sørensen type interaction [SorensenMolmer00], as in this example, but now in the off-resonant limit: \(\eta_i \Omega \ll |\nu_i-\delta|\), which gives:
Variable definitions:
\(\pmb{\Omega}\): Characterizes the strength of the ion-light interaction, which we assume to be identical for both lasers.
\(\pmb{\eta}\): Denotes the Lamb-Dicke factor, which characterizes the strength with which the laser interaction couples the ion’s motion to its electronic states.
\(\pmb{\delta}\): The two lasers, with frequencies \(\omega_i\), are detuned from the ion’s participating electronic transition frequency \(\omega_a\) as \(\omega_i-\omega_a=\pm\delta\).
Construct the system#
ca = Ca40([("S1/2", -1/2, "S"), ("D5/2", -1/2, "D")])
laser1 = Laser(pointing=[(1, 1.), (2, 1.)])
laser2 = Laser(pointing=[(1, 1.), (2, 1.)])
chain = LinearChain(
ions=[ca, ca],
comfrequencies=(x=3e6,y=3e6,z=1e6),
selectedmodes=(x=[1], z=[1]),
N=5 # Set Hilbert space dimension for participating vibrational modes
)
chamber = Chamber(iontrap=chain, B=6e-4, Bhat=(x̂ + ẑ)/√2, lasers=[laser1, laser2])
axial_mode = zmodes(chamber)[1]
radial_mode = xmodes(chamber)[1];
Set the laser parameters#
ϵ = 40e3
ν_axial = frequency(axial_mode)
wavelength_from_transition!(laser1, ca, ("S", "D"), chamber)
detuning!(laser1, ν_axial + ϵ)
polarization!(laser1, x̂)
wavevector!(laser1, ẑ)
wavelength_from_transition!(laser2, ca, ("S", "D"), chamber)
detuning!(laser2, -ν_axial - ϵ)
polarization!(laser2, x̂)
wavevector!(laser2, ẑ)
η = abs(lambdicke(axial_mode, ca, laser1))
Ω = √(1e3 * ϵ) / η # This will give a 1kHz MS strength, since coupling goes like (ηΩ)^2/ϵ
intensity_from_rabifrequency!(1, Ω, 1, ("S", "D"), chamber)
intensity_from_rabifrequency!(2, Ω, 1, ("S", "D"), chamber);
Construct Hamiltonian / Solve system#
h = hamiltonian(chamber, timescale=1e-6, lamb_dicke_order=1, rwa_cutoff=1e6);
@time tout, sol_res = timeevolution.schroedinger_dynamic(
0:2:3000, ca["D"] ⊗ ca["S"] ⊗ axial_mode[0] ⊗ radial_mode[0], h
);
44.166616 seconds (49.34 M allocations: 3.305 GiB, 3.95% gc time, 0.94% compilation time)
Plot results#
SD_res = expect(ionprojector(chamber, "S", "D"), sol_res)
plt.plot(tout, SD_res, label="resonant")
plt.xlim(tout[1], tout[end])
plt.ylim(0, 1)
plt.legend(loc=1)
plt.ylabel("SD")
plt.xlabel("Time (μs)");
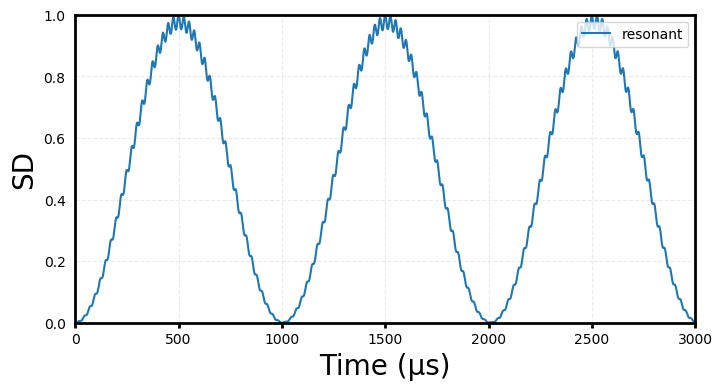
\(\hat{H}_{S-S} + \hat{H}_{S}\)#
Engineering the energy barrier#
\(\hat{H}_{S} = \frac{\Delta}{2} \hat{\sigma}_z^{(2)}\) can be engineered via an AC stark shift, B-field gradient or directly in IonSim with the stark_shift
field of an Ion
.
# We'll add the barrier term by setting a nonzero value to
# the trap's B-field gradient:
bgradient!(chamber, (1, 2), ("S", "D"), √5.25 * 1e3);
# ΩMS = 1 kHz, so setting Δ = √5.25 kHz gives Ω' = √(ΩMS^2 + Δ^2) = 2.5 kHz
Construct Hamiltonian / Solve system#
h = hamiltonian(chamber, timescale=1e-6, lamb_dicke_order=1, rwa_cutoff=1e6);
@time tout, sol_det = timeevolution.schroedinger_dynamic(
0:2:3000,
ca["D"] ⊗ ca["S"] ⊗ axial_mode[0] ⊗ radial_mode[0], h
);
1.505231 seconds (8.67 M allocations: 230.449 MiB, 12.53% gc time)
Plot results#
SD_det = expect(ionprojector(chamber, "S", "D"), sol_det)
plt.plot(tout, SD_res, label="resonant")
plt.plot(tout, SD_det, label="detuned")
plt.xlim(tout[1], tout[end])
plt.ylim(0, 1)
plt.legend(loc=1)
plt.ylabel("SD")
plt.xlabel("Time (μs)");
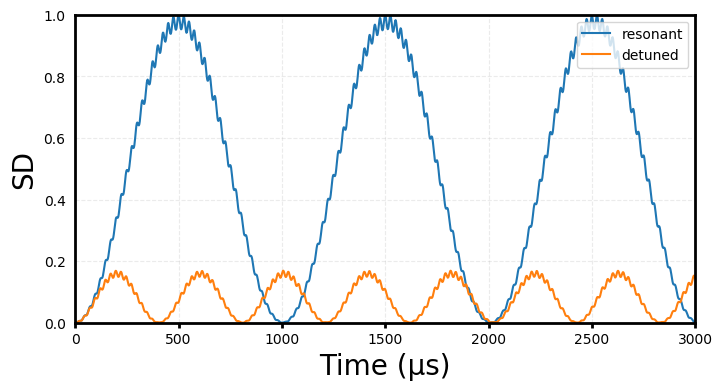
\(\hat{H}_{S-S} + \hat{H}_{S} + \hat{H}_{S-B} + \hat{H}_{B}\)#
Engineering the vibrational mode coupling#
The spin-bath coupling term can be engineered using two additional laser tones, in a way similar to the Mølmer Sørensen interaction, but shining on only a single ion and with the detunings from the carrier transition equal to plus/minus half of the frequency of the coupled vibrational mode []. This gives:
Here \(\Omega, \eta\) and \(\nu\) are defined as before, \(\hat{a}\) is the annihilation for the coupled vibrational mode and \(\nu_{eff}\) is controlled by a small asymmetry in the detunings of the two participating lasers (as described in [GHM+18]).
setup two additional lasers for the S-B Hamiltonian#
laser3 = Laser(pointing=[(2, 1.)])
laser4 = Laser(pointing=[(2, 1.)])
lasers!(chamber, [laser1, laser2, laser3, laser4]);
Set the parameters for these new lasers#
axial_mode = zmodes(chamber)[1]
radial_mode = xmodes(chamber)[1]
ν_radial = frequency(radial_mode)
νeff = √5.25 * 1e3
# We set the effective vibrational frequency by detuning
# the 2-photon transition off resonance
wavelength_from_transition!(laser3, ca, ("S", "D"), chamber)
detuning!(laser3, ν_radial / 2 + νeff)
polarization!(laser3, ẑ)
wavevector!(laser3, x̂)
wavelength_from_transition!(laser4, ca, ("S", "D"), chamber)
detuning!(laser4, -ν_radial / 2)
polarization!(laser4, ẑ)
wavevector!(laser4, x̂)
η = abs(lambdicke(radial_mode, ca, laser3))
Ω = √((0.5e3 * ν_radial) / η) # Set κ = 0.5 kHz, since κ = ηΩ^2/ν
intensity_from_rabifrequency!(3, Ω, 2, ("S", "D"), chamber)
intensity_from_rabifrequency!(4, Ω, 2, ("S", "D"), chamber);
Construct Hamiltonian / Solve system#
h = hamiltonian(chamber, timescale=1e-6, lamb_dicke_order=1, rwa_cutoff=1.51e6);
@time tout, sol_vaet = timeevolution.schroedinger_dynamic(
0:2:3000,
ca["D"] ⊗ ca["S"] ⊗ axial_mode[0] ⊗ radial_mode[0], h
);
87.876709 seconds (730.03 M allocations: 23.473 GiB, 9.04% gc time, 0.05% compilation time)
Plot results#
SD_vaet = expect(ionprojector(chamber, "S", "D"), sol_vaet)
plt.plot(tout, SD_res, label="resonant")
plt.plot(tout, SD_det, label="detuned")
plt.plot(tout, SD_vaet, label="VAET")
plt.xlim(tout[1], tout[end])
plt.ylim(0, 1)
plt.legend(loc=1)
plt.xlabel("Time (μs)");
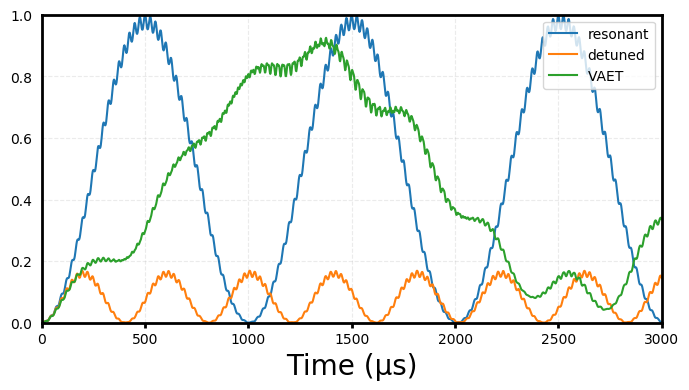
Bibliography#
- GHM+18(1,2)
Dylan J Gorman, Boerge Hemmerling, Eli Megidish, Soenke A. Moeller, Philipp Schindler, Mohan Sarovar, and Hartmut Haeffner. Engineering vibrationally assisted energy transfer in a trapped-ion quantum simulator. Phys. Rev. X, 8:011038, Mar 2018. URL: https://link.aps.org/doi/10.1103/PhysRevX.8.011038, doi:10.1103/PhysRevX.8.011038.